Claims Check Pattern: A Strategy for Handling Large Payloads in Distributed Systems
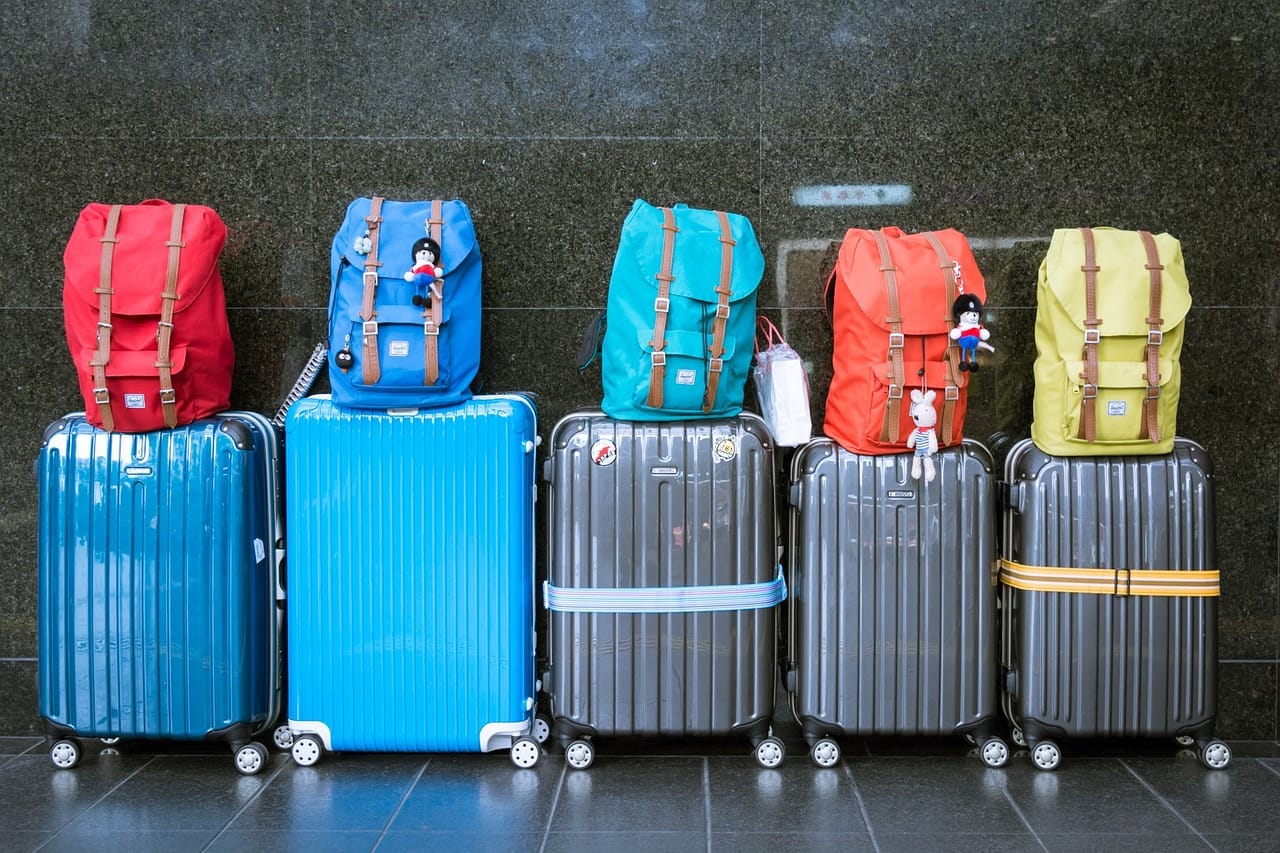
In modern distributed systems, managing the size of messages exchanged between services is critical for ensuring performance, scalability, and reliability. When dealing with large payloads, directly passing them between services can lead to issues such as increased network latency, memory consumption, and processing time. The Claims Check Pattern is a well-established design pattern that addresses these challenges by offloading large payloads to external storage and exchanging only references or "claims checks" between services.
In this blog post, we'll explore the Claims Check Pattern, discuss when and why to use it, and provide code snippets to demonstrate its implementation in a .NET environment.
What is the Claims Check Pattern?
The Claims Check Pattern is a design pattern used to handle large data payloads in messaging systems. Instead of passing the entire payload between services, the sender stores the payload in an external storage system (like a database, blob storage, or file system) and sends a lightweight message containing a reference (the "claims check") to the stored data. The receiver uses this reference to retrieve the data when needed.
This approach minimizes the size of messages exchanged between services, reducing the impact on network bandwidth, memory, and processing time.
When to Use the Claims Check Pattern
The Claims Check Pattern is beneficial in the following scenarios:
- Large Payloads: When the size of the data being exchanged exceeds the limits of your messaging system (e.g., queues or topics), or when large payloads negatively impact performance.
- Distributed Systems: In microservices architectures, where services communicate over the network and need to minimize message size to reduce latency and improve scalability.
- Scalability: When you need to ensure that your messaging infrastructure can handle high-throughput scenarios without being overwhelmed by large messages.
Implementing the Claims Check Pattern in .NET
Let's walk through a simple implementation of the Claims Check Pattern using Azure Blob Storage for external storage and Azure Service Bus for message passing. In this example, we'll assume we have a large document that needs to be processed by another service.
1. Storing the Payload and Sending the Claims Check
The sender service stores the large payload in Azure Blob Storage and sends a message containing the blob URL (the claims check) to Azure Service Bus.
public class SenderService
{
private readonly BlobServiceClient _blobServiceClient;
private readonly ServiceBusClient _serviceBusClient;
private readonly string _containerName = "documents";
public SenderService(BlobServiceClient blobServiceClient, ServiceBusClient serviceBusClient)
{
_blobServiceClient = blobServiceClient;
_serviceBusClient = serviceBusClient;
}
public async Task SendDocumentAsync(string documentId, byte[] documentData)
{
// Upload the document to Azure Blob Storage
var blobContainerClient = _blobServiceClient.GetBlobContainerClient(_containerName);
await blobContainerClient.CreateIfNotExistsAsync();
var blobClient = blobContainerClient.GetBlobClient($"{documentId}.pdf");
using var stream = new MemoryStream(documentData);
await blobClient.UploadAsync(stream, overwrite: true);
// Create a claims check message containing the blob URL
var claimsCheckMessage = new ServiceBusMessage(blobClient.Uri.ToString())
{
Subject = "DocumentProcessing"
};
// Send the claims check message to Azure Service Bus
var sender = _serviceBusClient.CreateSender("documentqueue");
await sender.SendMessageAsync(claimsCheckMessage);
}
}
2. Retrieving the Payload Using the Claims Check
The receiver service retrieves the blob URL from the message and uses it to download the document from Azure Blob Storage.
ublic class ReceiverService
{
private readonly BlobServiceClient _blobServiceClient;
private readonly ServiceBusClient _serviceBusClient;
public ReceiverService(BlobServiceClient blobServiceClient, ServiceBusClient serviceBusClient)
{
_blobServiceClient = blobServiceClient;
_serviceBusClient = serviceBusClient;
}
public async Task ProcessDocumentAsync()
{
var receiver = _serviceBusClient.CreateReceiver("documentqueue");
// Receive the claims check message from Azure Service Bus
ServiceBusReceivedMessage receivedMessage = await receiver.ReceiveMessageAsync();
string blobUrl = receivedMessage.Body.ToString();
// Download the document from Azure Blob Storage
var blobClient = new BlobClient(new Uri(blobUrl));
var blobDownloadInfo = await blobClient.DownloadAsync();
using var memoryStream = new MemoryStream();
await blobDownloadInfo.Value.Content.CopyToAsync(memoryStream);
// Process the document (for example, parse, analyze, etc.)
byte[] documentData = memoryStream.ToArray();
Console.WriteLine($"Processing document from {blobUrl} with size {documentData.Length} bytes");
// Complete the message processing
await receiver.CompleteMessageAsync(receivedMessage);
}
}
3. Handling Edge Cases
While the Claims Check Pattern is straightforward, it’s important to consider edge cases:
- Message Failure or Expiry: If the claims check message is lost or expires before being processed, the payload remains in storage without a reference. Implement a cleanup strategy to periodically delete orphaned blobs.
- Security Concerns: Ensure that the blob storage URL is secure, using Shared Access Signatures (SAS) to control access. Avoid exposing sensitive data via the claims check.
- Consistency: Ensure that the blob storage and messaging operations are consistent. If storing the blob succeeds but sending the message fails, the data could be lost. Implement retry logic and consider transactional messaging if available.
Conclusion
The Claims Check Pattern is a powerful tool for managing large payloads in distributed systems. By offloading the heavy lifting to external storage and passing lightweight references between services, you can improve performance, reduce network load, and ensure scalability. However, like any pattern, it’s important to handle edge cases carefully and secure the storage and retrieval process.
Whether you’re building microservices, event-driven architectures, or simply need to manage large messages, the Claims Check Pattern can help you design a more efficient and reliable system.