Creating Secret-less Services in the Cloud with Azure Managed Identities
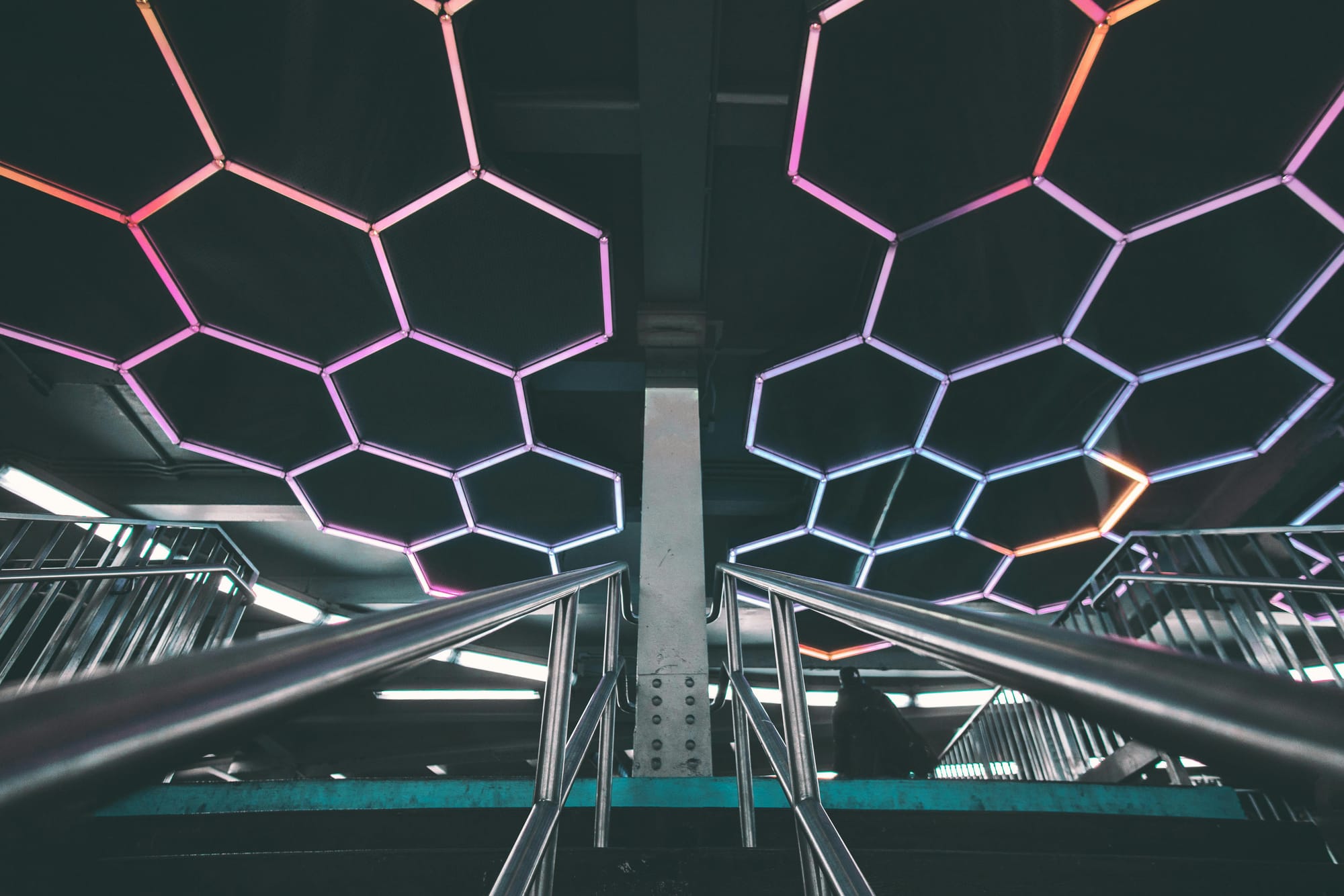
With the rise of secret-less architectures, you can reduce risk and simplify operations by eliminating hard-coded secrets in your applications. At Microsoft, we leverage this approach internally to ensure our own services remain secure and agile. In this blog post, we explore how to achieve secret-less services on Azure using Managed Identities, along with the underlying technology, security benefits, and best practices like Role-Based Access Control (RBAC) and Least Privilege Access (LPA).
The Need for Secret-less Architectures
Traditionally, developers store secrets in configuration files or environment variables, making them vulnerable to mismanagement or exposure. A secret-less design minimizes these risks by allowing applications to securely authenticate to cloud services without embedding credentials in code.
Azure Managed Identities provide an elegant solution to this problem. They enable your services to automatically acquire tokens from Azure Active Directory (Azure AD) without the need for secret management. This means your code remains cleaner, and you no longer need to worry about key rotations or accidental secret leakage.
How Managed Identities Work
Managed Identities are essentially identities managed by Azure AD that are automatically assigned to Azure resources (like Virtual Machines, App Services, or Functions). These identities help services authenticate securely without needing credentials stored in code or configuration.
When a resource with a Managed Identity needs to access another service (such as Azure Key Vault or Azure SQL Database), it requests an access token from Azure AD. Azure AD validates the resource's identity and issues a token, which the resource can then use to authenticate its requests. This flow is completely transparent to the developer, eliminating the need to manage any secrets manually.
Behind the Scenes
- Token Acquisition: Managed Identities use the OAuth 2.0 client credentials flow under the hood. The resource securely communicates with Azure AD and receives a token that includes its identity claims.
- Integration with Azure Services: Once the token is obtained, it can be used to access any Azure resource that supports Azure AD authentication. For instance, accessing Azure Key Vault or connecting to an Azure SQL Database.
- Automatic Key Rotation: Because the tokens are short-lived and managed by Azure AD, you don’t have to worry about token expiration or secret rotation.
Security Benefits: RBAC and LPA
Role-Based Access Control (RBAC)
Azure RBAC is a key element in securing your resources. With RBAC, you can grant fine-grained access permissions to resources based on roles. This means that even if an application has a Managed Identity, it will only be able to perform actions that its role explicitly allows. For example, you might assign a "Key Vault Reader" role to an app that only needs to retrieve secrets from Key Vault.
Least Privilege Access (LPA)
LPA is the practice of granting only the minimum permissions necessary to perform a task. By combining RBAC with Managed Identities, you ensure that your services operate under the principle of least privilege. This minimizes the impact of any potential compromise since each service can only access the resources it genuinely needs.
Implementing Secret-less Services in C#
Let’s take a look at some practical C# examples that demonstrate how to leverage Azure Managed Identities.
Example 1: Accessing Azure Key Vault
This example shows how to use the DefaultAzureCredential
class from the Azure Identity library to access a secret stored in Azure Key Vault:
namespace SecretLessApp
{
class Program
{
static async Task Main(string[] args)
{
var keyVaultUrl = "https://your-keyvault-name.vault.azure.net/";
// Create a SecretClient using DefaultAzureCredential which supports Managed Identities
var client = new SecretClient(new Uri(keyVaultUrl), new DefaultAzureCredential());
KeyVaultSecret secret = await client.GetSecretAsync("MySecret");
}
}
}
This code uses the Managed Identity assigned to your Azure resource to obtain an access token for Azure Key Vault, ensuring that no secrets are hard-coded in your application.
Example 2: Connecting to Azure SQL Database with Managed Identity
For connecting to an Azure SQL Database using Managed Identity, you can configure your connection string to leverage Azure AD authentication:
namespace SecretLessSqlApp
{
class Program
{
static void Main(string[] args)
{
// Connection string with Managed Identity authentication
var connectionString = "Server=tcp:yourserver.database.windows.net,1433;" +
"Database=yourdatabase;" +
"Authentication=Active Directory Managed Identity;";
using (var connection = new SqlConnection(connectionString))
{
connection.Open();
Console.WriteLine("Connection established successfully using Managed Identity.");
// Execute SQL queries as needed...
}
}
}
}
In this example, the connection to Azure SQL Database is authenticated via the Managed Identity, removing the need to manage and store SQL credentials.
Adopting a secret-less approach in your cloud services can significantly enhance your security posture while simplifying your codebase. Azure Managed Identities provide a robust mechanism to authenticate securely without the overhead of secret management. When combined with RBAC and the principles of Least Privilege Access, you can ensure that your applications have only the access they need, thereby reducing the potential attack surface.
At Microsoft, we leverage this approach internally to build secure, scalable, and maintainable services—an approach that can be applied to your projects as well. By embracing these strategies, you’re well on your way to building more secure, maintainable, and scalable cloud applications.