Exploring Mutation Testing
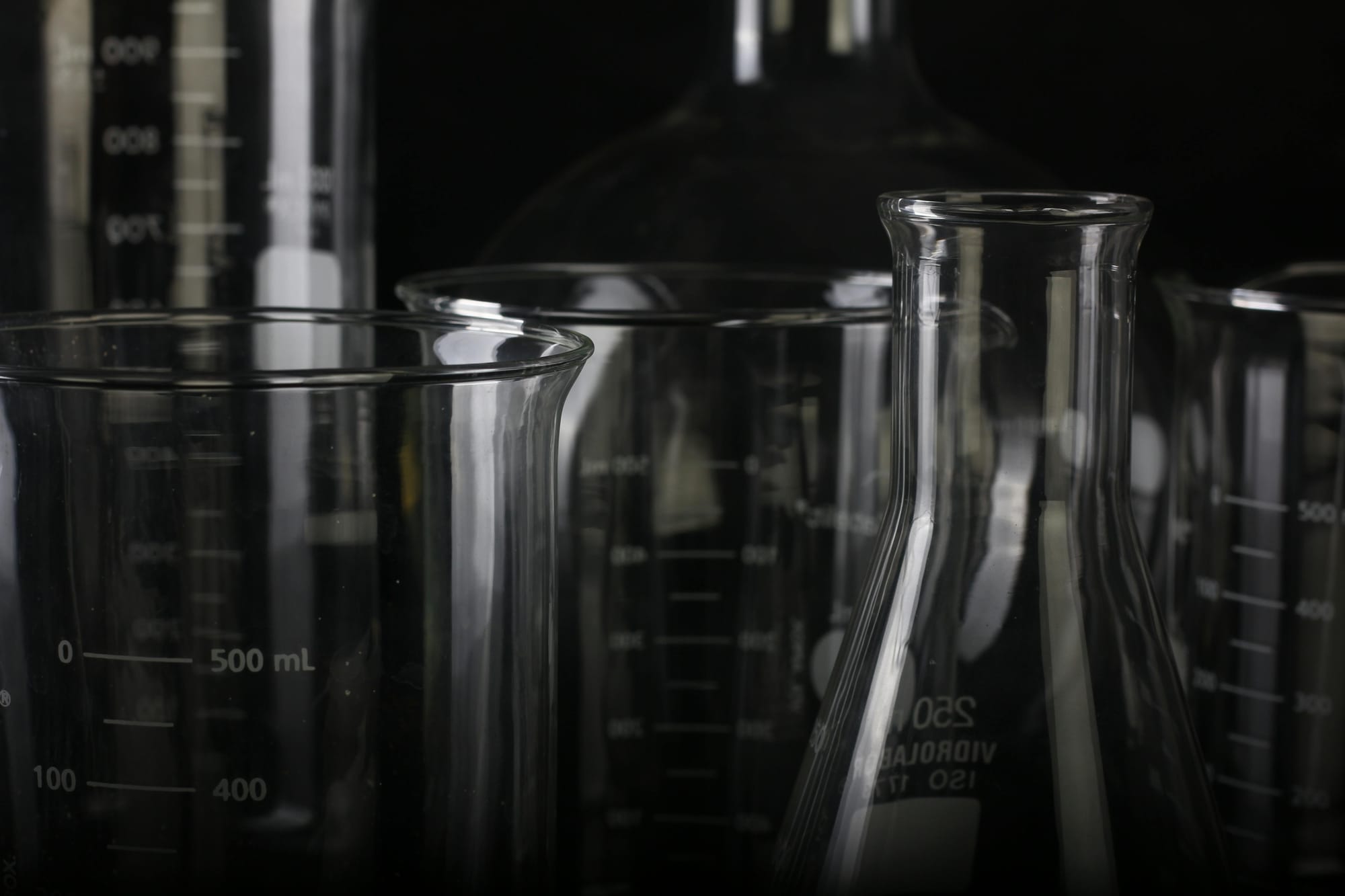
Testing is an essential part of software development, ensuring code behaves as expected and remains robust in the face of change. But how do you know if your tests are truly effective? Enter mutation testing, a technique that goes beyond traditional code coverage metrics to evaluate the quality of your test suite.
What is Mutation Testing?
Mutation testing involves deliberately introducing small changes, or "mutations," to your codebase and then running your tests to see if they catch the errors. These mutations mimic common coding mistakes, such as flipping a conditional, changing an operator, or altering a constant.
If your tests detect and fail due to the mutation, the mutant is killed. If they pass despite the mutation, the mutant survives, indicating a gap in your test coverage.
Why Use Mutation Testing?
Traditional metrics like code coverage only tell you how much of your code was executed during testing—they don’t measure whether your tests actually verify the correctness of the code. Mutation testing addresses this by assessing:
- Test strength: Are your tests robust enough to catch errors?
- Coverage quality: Do your tests focus on verifying important behavior?
By focusing on these aspects, mutation testing helps ensure your test suite isn't just touching code but genuinely validating it.
A Simple Example
Consider the following Java code:
public class Calculator {
public int add(int a, int b) {
return a + b;
}
}
And a corresponding test:
import static org.junit.jupiter.api.Assertions.assertEquals;
import org.junit.jupiter.api.Test;
class CalculatorTest {
@Test
void testAddition() {
Calculator calculator = new Calculator();
assertEquals(5, calculator.add(2, 3));
}
}
Mutation testing might introduce a change like flipping the +
operator to -
in the add
method. If the test still passes, it indicates the test doesn’t adequately verify the behavior of the add
method.
Tools for Mutation Testing
There are several tools available to help automate mutation testing. Popular ones include:
- PIT (Java)
- MutPy (Python)
- Stryker (JavaScript/TypeScript, .NET, Python, etc.)
These tools generate mutants, run your tests, and provide detailed reports on survivors and killed mutants.
Challenges of Mutation Testing
While powerful, mutation testing isn't without its challenges:
- Performance: Running tests against every mutation can be time-consuming for large projects.
- False positives: Some mutants may survive due to limitations in the mutation logic rather than test quality.
- Analysis overhead: Reviewing mutation reports requires time and effort to identify genuine gaps in coverage.
Conclusion
Mutation testing provides a deeper understanding of your test suite’s effectiveness, helping ensure that your tests truly safeguard your codebase. While it may require additional setup and resources, the insights it offers can lead to more robust and maintainable software.