OO & Functional Programming, Blending Paradigms
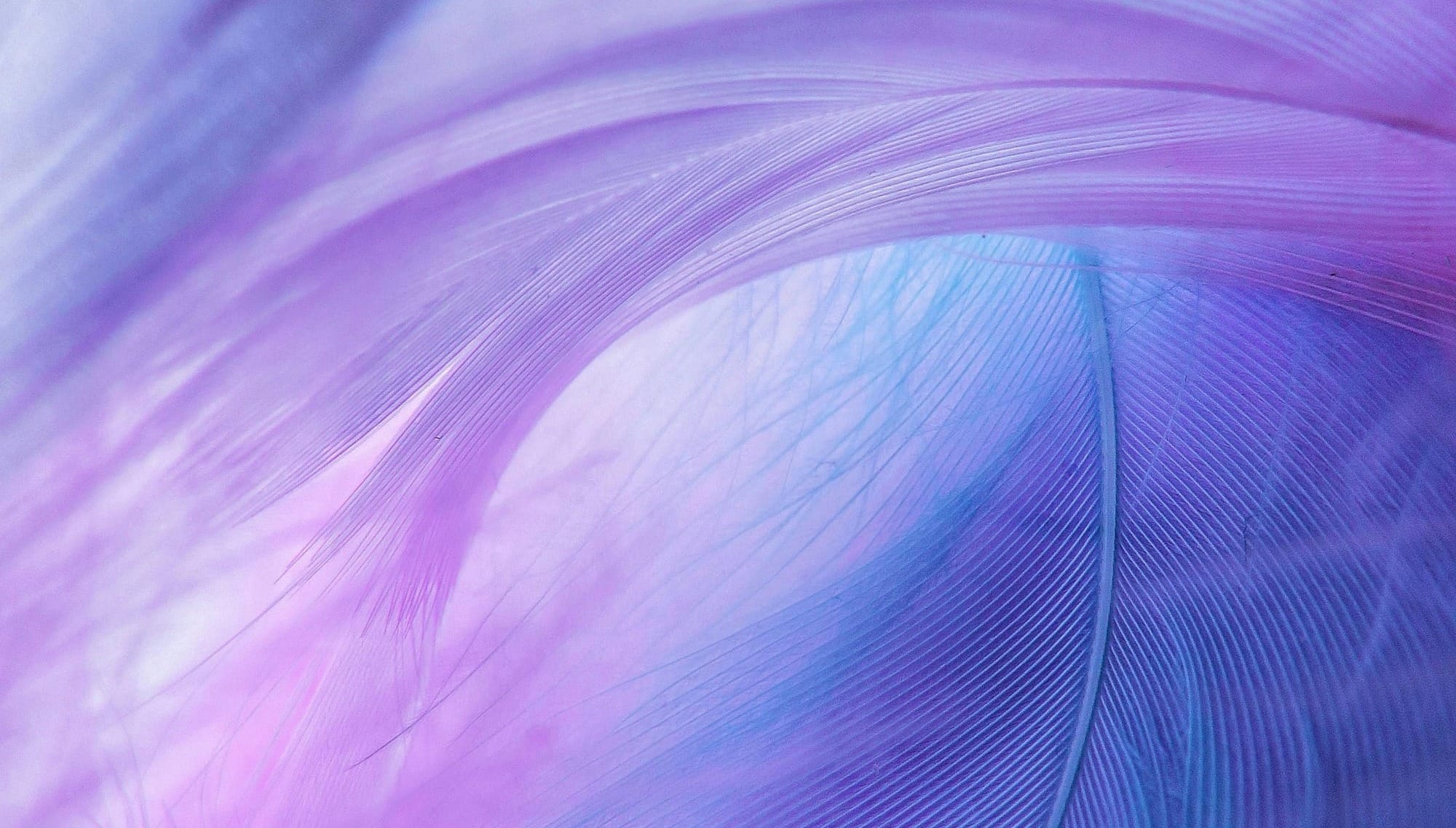
In software development, the lines between programming paradigms are becoming increasingly blurred. Traditionally, object-oriented (OO) programming and functional programming have been seen as distinct, almost opposing, approaches. However, languages like C# are evolving into multi-paradigm powerhouses that let us harness the strengths of both worlds. By combining OO concepts such as encapsulation, inheritance, and polymorphism with functional ideas like immutability, higher-order functions, and lambda expressions, we can create code that is both robust and expressive.
At its core, object-oriented programming helps us model our domain with objects that encapsulate state and behaviour. Functional programming, on the other hand, focuses on composing small, pure functions to build more complex behavior. When you merge these paradigms, you can enjoy benefits such as easier reasoning about state changes, improved testability, and enhanced modularity.
One way to blend these paradigms is by using immutable objects. In an OO system, objects often maintain mutable state, which can lead to hidden side effects. By designing immutable classes, you get many of the benefits of functional programming. Consider this simple example in C# where we define an immutable point.
public class Point
{
public int X { get; }
public int Y { get; }
public Point(int x, int y)
{
X = x;
Y = y;
}
public Point Move(int deltaX, int deltaY)
{
return new Point(X + deltaX, Y + deltaY);
}
public override string ToString() => $"Point({X}, {Y})";
}
In this design, any operation that “changes” the state of the object instead returns a new object. This approach makes our code easier to understand and debug, especially in concurrent or asynchronous contexts where mutable state can lead to subtle bugs.
Another powerful aspect of C# is its support for higher-order functions and lambda expressions. By integrating these functional constructs into our OO code, we can create flexible and reusable components. For instance, imagine you have a collection of points and you want to filter them based on a custom condition. You can encapsulate the filtering logic as a lambda expression.
public class PointProcessor
{
public IEnumerable<Point> FilterPoints(IEnumerable<Point> points, Func<Point, bool> predicate)
{
return points.Where(predicate);
}
}
var points = new List<Point>
{
new Point(1, 2),
new Point(3, 4),
new Point(5, 6)
};
var processor = new PointProcessor();
var filteredPoints = processor.FilterPoints(points, point => point.X > 2);
foreach (var point in filteredPoints)
{
Console.WriteLine(point);
}
In this snippet, the FilterPoints
method accepts a Func<Point, bool>
delegate that represents the filtering criteria. This design allows clients of the class to supply custom logic without needing to subclass or modify the PointProcessor
. It’s a great example of how higher-order functions can lead to more abstract and decoupled designs.
Another opportunity for merging OO and functional paradigms is found in the use of extension methods. These methods let you “extend” the functionality of existing classes in a functional style. For example, consider adding a method to transform a point using a provided function.
public static class PointExtensions
{
public static Point Transform(this Point point, Func<Point, Point> transformer)
{
return transformer(point);
}
}
var originalPoint = new Point(2, 3);
var transformedPoint = originalPoint.Transform(p => p.Move(5, -1));
Console.WriteLine(transformedPoint);
With extension methods, you can write fluent, chainable code that reads almost like a natural language. This greatly improves the expressiveness of your code and encourages the use of small, composable functions.
Moreover, the interplay of OO and functional programming shines when you design domain models. For example, you might create a class hierarchy to represent various shapes, and then use functional techniques to operate on these objects without exposing their internal state. Imagine a base class Shape
with derived classes Circle
and Rectangle
.
public abstract class Shape
{
public abstract double Area();
}
public class Circle : Shape
{
public double Radius { get; }
public Circle(double radius)
{
Radius = radius;
}
public override double Area() => Math.PI * Radius * Radius;
}
public class Rectangle : Shape
{
public double Width { get; }
public double Height { get; }
public Rectangle(double width, double height)
{
Width = width;
Height = height;
}
public override double Area() => Width * Height;
}
Now, suppose you want to calculate the total area of a collection of shapes. You can apply functional programming techniques like aggregation.
using System.Collections.Generic;
using System.Linq;
public static class ShapeCalculator
{
public static double TotalArea(IEnumerable<Shape> shapes)
{
return shapes.Select(shape => shape.Area()).Sum();
}
}
// Usage example:
var shapes = new List<Shape>
{
new Circle(5),
new Rectangle(4, 6)
};
double totalArea = ShapeCalculator.TotalArea(shapes);
Console.WriteLine($"Total Area: {totalArea}");
In this design, the OO principles help structure the domain model, while the functional approach simplifies operations on collections of objects.
Combining OO and functional programming in C# isn’t about choosing one over the other—it’s about leveraging both paradigms to write cleaner, more maintainable code. Embracing immutable objects minimizes side effects, while higher-order functions and lambda expressions promote flexibility and modularity. Extension methods further enhance your ability to write fluent code that seamlessly blends OO structures with functional transformations.
By recognizing and embracing the strengths of both paradigms, developers can craft solutions that are resilient, easy to test, and scalable. Whether you are refactoring legacy code or building a new application from scratch, consider how these combined approaches can lead to better software design. The evolution of C# into a multi-paradigm language offers a rich toolkit to meet modern development challenges.