The Reservation Pattern: A Strategy for Handling Distributed Transactions
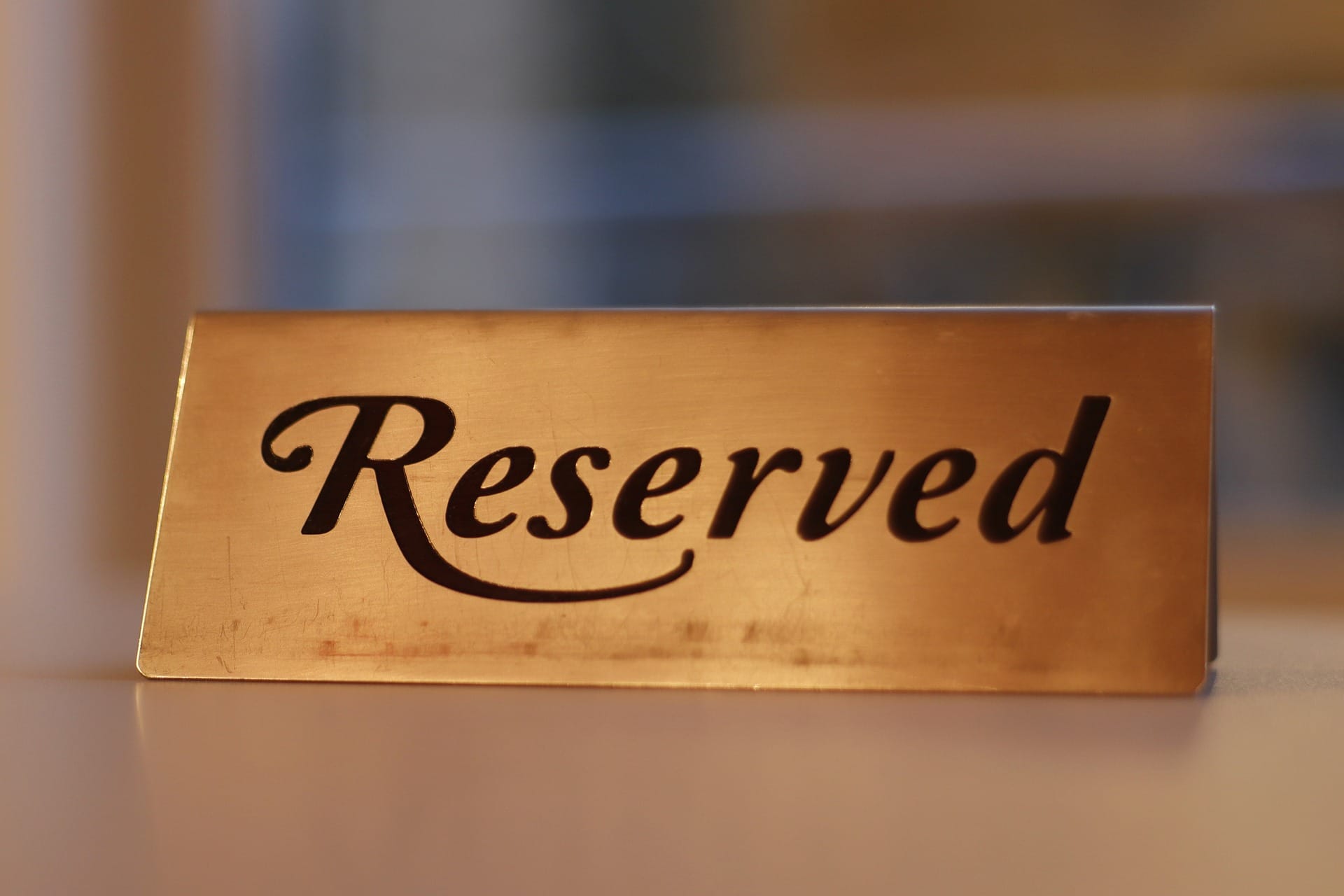
In distributed systems, ensuring data consistency across multiple services can be challenging, especially when dealing with scenarios that require eventual consistency or involve booking, allocation, or reservations. The Reservation Pattern is a useful strategy for managing these types of transactions, where resources must be reserved and confirmed before finalizing a transaction. This pattern is particularly effective in preventing race conditions, double bookings, or over-allocations in a distributed environment.
What is the Reservation Pattern?
The Reservation Pattern involves a two-step process:
- Reservation: A resource or item is reserved for a limited time, making it unavailable to others during this period. This reservation does not immediately finalize the transaction but holds the resource to ensure it is available when the transaction is completed.
- Confirmation: If the transaction proceeds, the reservation is confirmed, making the allocation permanent. If the transaction does not complete, the reservation is released, allowing others to reserve or allocate the resource.
This pattern is commonly used in scenarios like hotel bookings, inventory management, ticketing systems, and more.
Implementing the Reservation Pattern
Here’s how you might implement the Reservation Pattern in a distributed system:
- Create a Reservation:
When a user requests a resource, you create a reservation. This reservation has a unique identifier, the resource details, and an expiration time (e.g., 10 minutes). The resource is marked as "reserved" and is temporarily unavailable for other transactions.
public Reservation CreateReservation(Resource resource, User user)
{
var reservation = new Reservation
{
Id = Guid.NewGuid(),
ResourceId = resource.Id,
UserId = user.Id,
ExpirationTime = DateTime.UtcNow.AddMinutes(10)
};
resource.Status = ResourceStatus.Reserved;
_reservationRepository.Add(reservation);
return reservation;
}
- Confirm the Reservation:
If the user completes the transaction (e.g., checks out or finalizes the booking), the reservation is confirmed. The resource is then marked as "allocated" or "sold," and the reservation is removed or marked as completed.
public void ConfirmReservation(Guid reservationId)
{
var reservation = _reservationRepository.GetById(reservationId);
if (reservation != null && reservation.ExpirationTime > DateTime.UtcNow)
{
var resource = _resourceRepository.GetById(reservation.ResourceId);
resource.Status = ResourceStatus.Allocated;
_reservationRepository.Remove(reservation);
}
else
{
throw new InvalidOperationException("Reservation has expired or is invalid.");
}
}
- Handle Expiration:
If the reservation is not confirmed within the expiration time, it automatically expires, and the resource becomes available again. This can be managed with a background process that periodically checks for expired reservations and releases them.
public void ExpireReservations()
{
var expiredReservations = _reservationRepository.GetExpiredReservations(DateTime.UtcNow);
foreach (var reservation in expiredReservations)
{
var resource = _resourceRepository.GetById(reservation.ResourceId);
resource.Status = ResourceStatus.Available;
_reservationRepository.Remove(reservation);
}
}
Edge Cases and Considerations
While the Reservation Pattern is powerful, there are several edge cases to consider:
- Double Booking:
- If the system does not check availability before creating a reservation, it can lead to double bookings. Ensure that the resource’s status is consistently checked before reserving it.
- Expiration Timing:
- If a transaction takes longer than expected to complete, the reservation might expire prematurely. Carefully choose expiration times, and consider user behavior patterns when setting them.
- Partial Failures:
- In distributed systems, partial failures can occur, such as the payment system failing after the reservation is confirmed. Implement compensating actions, like rolling back the confirmation if payment fails.
- Concurrency Issues:
- Ensure that your reservation and confirmation logic handles concurrency correctly. Use appropriate locking mechanisms or transactions to prevent race conditions.
- Scalability:
- In high-traffic scenarios, consider the scalability of your reservation system. Using distributed caches or databases with strong consistency guarantees can help manage large volumes of reservations.
Conclusion
The Reservation Pattern is a valuable approach for managing resources in distributed systems where data consistency and availability are critical. By separating the reservation and confirmation steps, you can handle complex scenarios like bookings, allocations, and purchases more reliably. However, it’s essential to be aware of the potential edge cases and ensure that your implementation can handle them gracefully.
By thoughtfully applying the Reservation Pattern, you can build more resilient and user-friendly systems that avoid common pitfalls like double bookings or over-allocations.