Understanding Cyclomatic Complexity
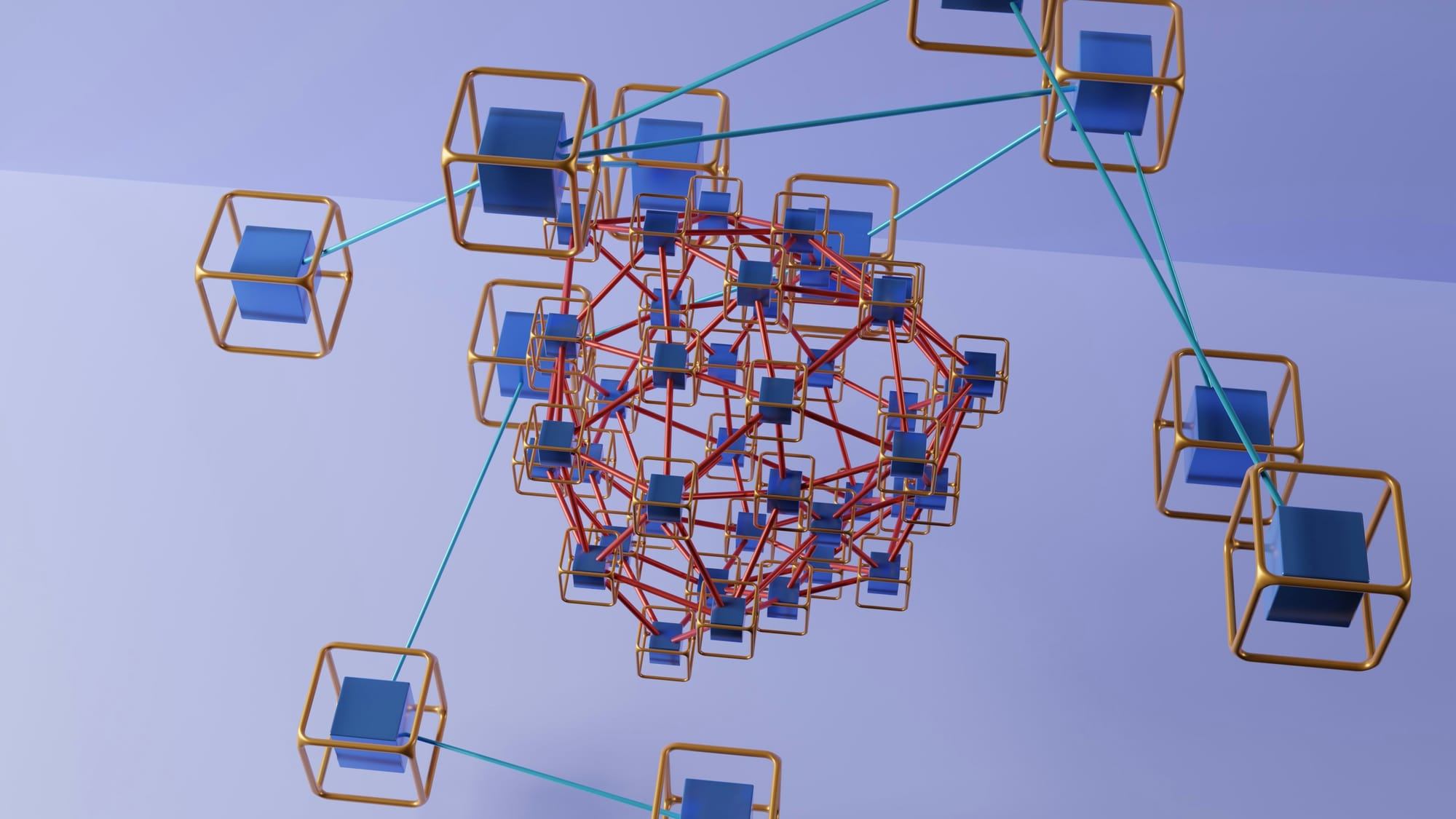
When we develop, we all aim to write clean, efficient, and maintainable code. One metric that helps measure the complexity of our code is Cyclomatic Complexity. While it sounds intimidating, understanding and managing it can significantly improve your code quality.
What Is Cyclomatic Complexity?
Cyclomatic Complexity is a software metric that measures the number of independent paths through a program’s source code. In simpler terms, it quantifies the complexity of a program based on its control flow—things like loops, conditionals, and case statements.
Here’s the formula for Cyclomatic Complexity:
- E: Number of edges (transitions between code blocks)
- N: Number of nodes (code blocks)
- P: Number of connected components (typically 1 for a single function)
The higher the Cyclomatic Complexity, the more complex the code, making it harder to test, maintain, and debug.
Why Does Cyclomatic Complexity Matter?
High Cyclomatic Complexity can lead to:
- Hard-to-maintain code: Complex functions are challenging to understand and modify.
- Increased testing effort: More paths mean more test cases are needed for thorough coverage.
- Bug-prone code: Complex logic is more likely to harbor defects.
On the flip side, low Cyclomatic Complexity often indicates simpler, more maintainable code.
Calculating Cyclomatic Complexity
Let’s consider a simple Java example:
Example 1: Simple Function
public boolean isEven(int number) {
return number % 2 == 0;
}
This function has:
- 1 node (the return statement)
- 0 edges (no decision points)
Cyclomatic Complexity: 1
Example 2: Conditional Logic
public String classifyNumber(int number) {
if (number > 0) {
return "Positive";
} else if (number < 0) {
return "Negative";
} else {
return "Zero";
}
}
Here we have:
- 4 nodes (if, else if, else, and the return statements)
- 3 edges (decisions to each branch)
Cyclomatic Complexity: 3
Managing Cyclomatic Complexity
Refactor Large Methods
When a function has high Cyclomatic Complexity, it’s often a sign that it does too much. Break it down into smaller, more focused methods.
Before: High Complexity
public String processOrder(Order order) {
if (order.isPaid()) {
if (order.isShipped()) {
return "Order Complete";
} else {
return "Awaiting Shipment";
}
} else {
return "Payment Pending";
}
}
Cyclomatic Complexity: 4
After: Refactored
public String processOrder(Order order) {
if (!order.isPaid()) {
return "Payment Pending";
}
return order.isShipped() ? "Order Complete" : "Awaiting Shipment";
}
Cyclomatic Complexity: 3
Use Polymorphism or Strategy Pattern
High complexity often comes from multiple conditional branches. Polymorphism or a strategy pattern can simplify such cases.
Before: Complex Conditional Logic
public double calculateDiscount(Customer customer) {
if (customer.isPremium()) {
return 0.2;
} else if (customer.isLoyal()) {
return 0.1;
} else {
return 0.05;
}
}
Cyclomatic Complexity: 3
After: Using Polymorphism
public interface DiscountStrategy {
double getDiscount();
}
public class PremiumDiscount implements DiscountStrategy {
public double getDiscount() {
return 0.2;
}
}
public class LoyalDiscount implements DiscountStrategy {
public double getDiscount() {
return 0.1;
}
}
public class RegularDiscount implements DiscountStrategy {
public double getDiscount() {
return 0.05;
}
}
// Usage
DiscountStrategy strategy = customer.getDiscountStrategy();
return strategy.getDiscount();
yclomatic Complexity per class: 1
Tools to Measure Cyclomatic Complexity
There are many tools to calculate Cyclomatic Complexity in Java:
- SonarQube: A popular static analysis tool that reports complexity metrics.
- JaCoCo: Measures code coverage and can provide insights into complexity.
- IntelliJ IDEA: Offers built-in inspection for Cyclomatic Complexity.
When I first encountered Cyclomatic Complexity, it felt like an abstract metric that didn’t quite connect with real-world problems. But over time, I’ve seen how managing complexity makes code easier to read, test, and maintain. Every time I refactor a messy method, I’m reminded of the value of simplicity.
Remember, Cyclomatic Complexity is a guide, not a hard rule. It’s okay for some functions to have higher complexity if they’re naturally intricate, but aim to keep most of your code straightforward.