Understanding the Law of Demeter
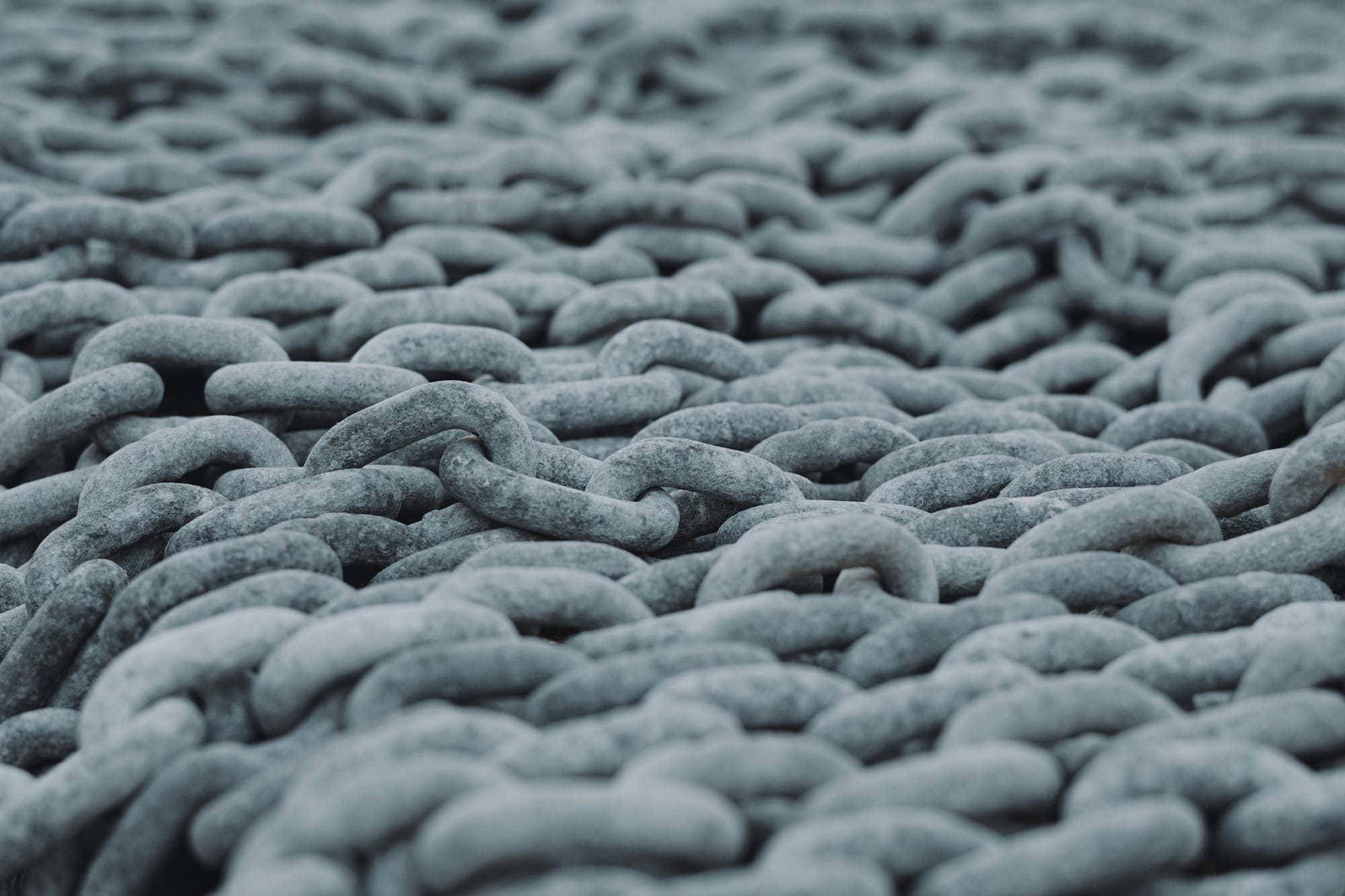
The Law of Demeter (LoD), also known as the "Principle of Least Knowledge," is a software design guideline for improving the modularity and maintainability of your code. At its core, the rule advises: "An object should only interact with its direct collaborators."
In simpler terms, an object should "talk to friends, not strangers." This means you should avoid chaining method calls or accessing deeply nested objects within your code.
Let’s dive into why this principle matters and see examples in Java to illustrate the concept.
The Problem: Breaking the Law of Demeter
When we violate the Law of Demeter, our code becomes tightly coupled, harder to maintain, and difficult to test. Here's an example:
class Engine {
public void start() {
System.out.println("Engine started.");
}
}
class Car {
private Engine engine;
public Car(Engine engine) {
this.engine = engine;
}
public Engine getEngine() {
return engine;
}
}
class Driver {
public void startCar(Car car) {
// Violation: Accessing the Engine object through Car
car.getEngine().start();
}
}
In this code:
- The
Driver
class interacts with theCar
object. - It directly calls the
getEngine()
method to access theEngine
object and then invokes thestart()
method.
This chain of calls (car.getEngine().start()
) violates the Law of Demeter, as Driver
now depends on the internal structure of Car
.
The Solution: Adhering to the Law of Demeter
To follow the Law of Demeter, we should ensure that objects only interact with their immediate collaborators. Here's how we can fix the above example:
class Engine {
public void start() {
System.out.println("Engine started.");
}
}
class Car {
private Engine engine;
public Car(Engine engine) {
this.engine = engine;
}
public void start() {
engine.start(); // Car delegates the start action to Engine
}
}
class Driver {
public void startCar(Car car) {
// Adheres to LoD: Directly interacting with Car
car.start();
}
}
In this refactored version:
- The
Car
class encapsulates theEngine
object and provides astart()
method. - The
Driver
class no longer interacts withEngine
directly, improving encapsulation and reducing coupling.
Why the Law of Demeter Matters
Adhering to the Law of Demeter has several benefits:
- Improved Maintainability: Changes in one class are less likely to ripple through others.
- Easier Testing: Reduced coupling means individual classes can be tested independently.
- Enhanced Readability: Code is more intuitive and easier to understand.
Practical Takeaways
While the Law of Demeter is a useful guideline, it’s not a hard rule. Over-applying it can lead to unnecessary boilerplate code (e.g., adding too many delegation methods). Use your judgment to balance encapsulation with simplicity.