Understanding the Result Pattern: Enhancing Code Quality and Error Handling
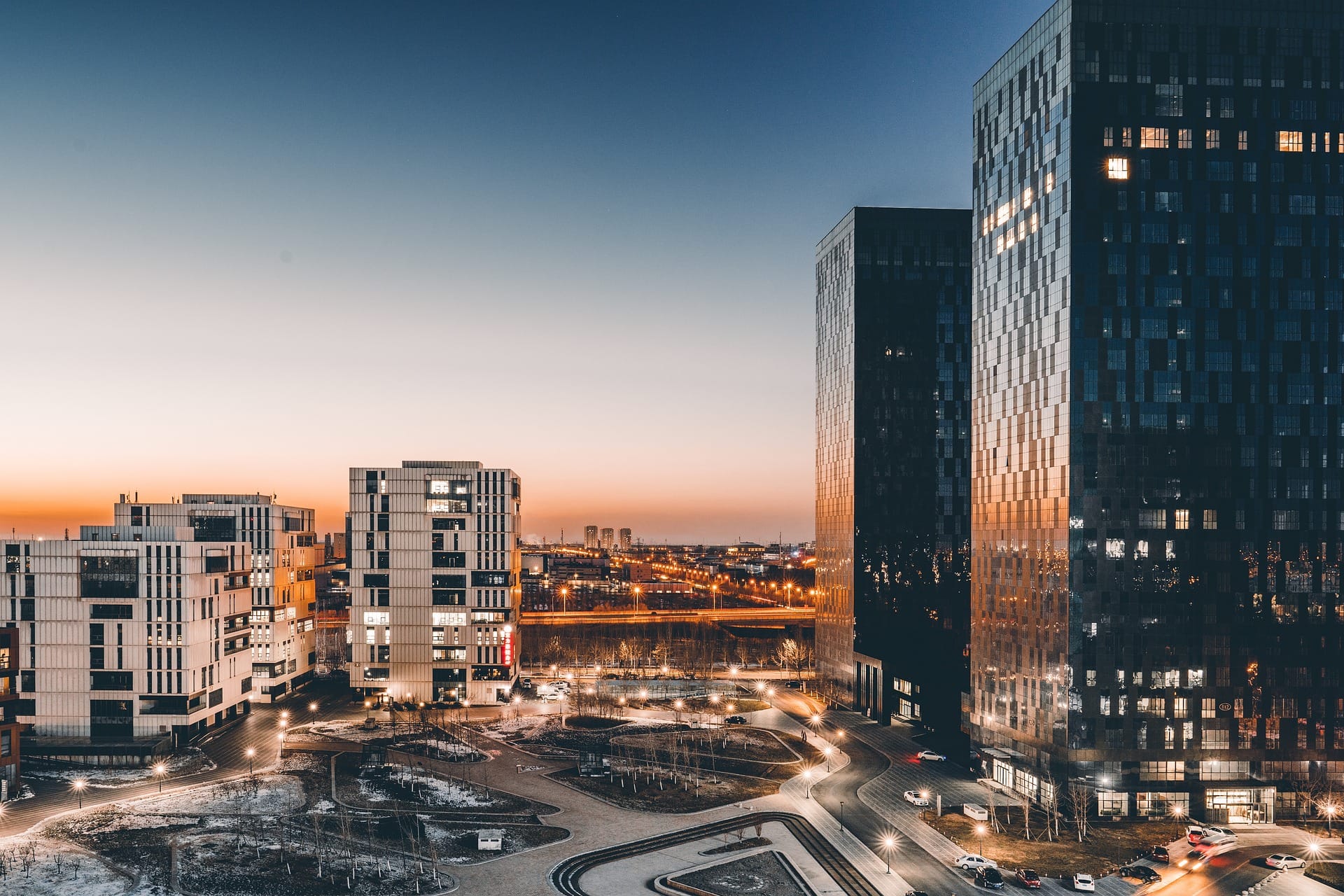
When developing software, handling errors and managing the results of operations is a crucial aspect of writing reliable and maintainable code. One approach to this is using the Result pattern, which is becoming increasingly popular in modern C# development. The Result pattern allows you to encapsulate the outcome of an operation, including both its success or failure state, and any associated data or error messages. This blog post explores the Result pattern, why it’s beneficial, and how it can be implemented in C#.
What is the Result Pattern?
The Result pattern is a design pattern used to represent the outcome of an operation that can either succeed or fail. Instead of relying on exceptions for error handling, the Result pattern provides a more explicit and functional way to manage both successful and failed operations. This pattern typically includes:
- Success State: The operation was successful, and the result (if any) is returned.
- Failure State: The operation failed, and an error message or code is provided.
Using the Result pattern, developers can handle these two states explicitly, avoiding common pitfalls like unhandled exceptions or ambiguous return values.
Benefits of the Result Pattern
- Explicit Error Handling: Unlike traditional exception handling, the Result pattern makes error handling explicit. This reduces the risk of unhandled exceptions and ensures that developers consider failure cases.
- Improved Readability: The Result pattern provides clear and readable code, making it easier to understand the flow of the application, especially when dealing with multiple operations that could fail.
- Better Control Flow: By handling success and failure cases separately, developers can create more robust and maintainable code. The Result pattern also helps avoid deeply nested if-else statements, leading to cleaner code.
- Encapsulation of Errors: The Result pattern encapsulates errors within a single object, making it easier to pass errors around without losing context or needing to rely on exceptions.
Implementing the Result Pattern in C#
Below is an implementation of the Result pattern in C#. This example provides a Result
class that represents the outcome of an operation and a Result<TValue>
class that represents the outcome when the operation produces a value.
public class Result
{
protected static readonly Error None = new(ErrorCode.None, "There is no error.");
protected static readonly Error NullValue = new(ErrorCode.NullValue, "The specified result value is null.");
protected internal Result(bool isSuccess, Error error)
{
if (isSuccess && error != None)
Throw.InvalidOperationException("A successful result cannot have an associated error.");
if (!isSuccess && error == None)
Throw.InvalidOperationException("A failed result must have an associated error.");
IsSuccess = isSuccess;
Error = error;
}
public bool IsSuccess { get; }
public bool IsFailure => !IsSuccess;
public Error Error { get; }
public static Result Success() => new(true, None);
public static Result Failure(Error error)
{
Throw.IfNull(error);
return new(false, error);
}
public static Result<TValue> Success<TValue>(TValue value) => new(true, None, value);
public static Result<TValue> Failure<TValue>(Error error) => new(false, error, default);
public static Result<TValue> Create<TValue>(TValue? value) => value is not null ? Success(value) : Failure<TValue>(NullValue);
public TResult Match<TResult>(Func<TResult> onSuccess, Func<Error, TResult> onFailure) => IsSuccess ? onSuccess() : onFailure(Error);
public void OnSuccess(Action onSuccess)
{
if (IsSuccess)
onSuccess();
}
public void OnFailure(Action<Error> onFailure)
{
if (IsFailure)
onFailure(Error);
}
public void OnBoth(Action onSuccess, Action<Error> onFailure)
{
if (IsSuccess)
onSuccess();
else
onFailure(Error);
}
public override string ToString() =>
IsSuccess ? "Success" : $"Failure: ErrorCode = {Error.Code}, Message = {Error.Message}";
}
public class Result<TValue> : Result
{
public TValue? Value { get; }
protected internal Result(bool isSuccess, Error error, TValue? value)
: base(isSuccess, error)
{
Value = value;
}
public static Result<TValue> Success(TValue value)
{
Throw.IfNull(value);
return new Result<TValue>(true, None, value);
}
public bool TryGetValue(out TValue? value)
{
value = Value;
return IsSuccess;
}
public void OnBoth(Action<TValue?> onResult, Action<Error> onError)
{
if (IsSuccess)
onResult(Value);
else
onError(Error);
}
public static implicit operator Result<TValue>(TValue? value) => Create(value);
public override string ToString() =>
IsSuccess
? $"Success: Value Type = {Value?.GetType().Name ?? "null"}, Value = {Value}"
: $"Failure: ErrorCode = {Error.Code}, Message = {Error.Message}";
}
How to Use the Result Pattern
The Result pattern can be used in various scenarios, such as validating inputs, handling database operations, or processing external API calls. Here’s an example of how you might use the Result
and Result<T>
classes:
public Result ProcessOrder(Order order)
{
if (order == null)
return Result.Failure(new Error(ErrorCode.NullValue, "Order cannot be null."));
if (order.Items.Count == 0)
return Result.Failure(new Error(ErrorCode.Validation, "Order must contain at least one item."));
// Process order...
return Result.Success();
}
public Result<string> GenerateInvoice(Order order)
{
if (order == null)
return Result<string>.Failure(new Error(ErrorCode.NullValue, "Order cannot be null."));
var invoiceId = Guid.NewGuid().ToString();
return Result<string>.Success(invoiceId);
}
In this example, the ProcessOrder
method returns a Result
indicating whether the operation was successful, while GenerateInvoice
returns a Result<string>
that includes a generated invoice ID if successful.
Wrapping Up
The Result pattern is a powerful and expressive way to handle success and failure in your C# applications. By explicitly managing both outcomes, it encourages better error handling, improves code readability, and reduces the risk of unexpected errors. Whether you’re building small utilities or large enterprise systems, the Result pattern can help you write cleaner, more reliable code.
Adopting the Result pattern in your projects can lead to more robust applications, where error conditions are handled gracefully, and success states are managed clearly. If you’re looking to improve your approach to error handling in C#, give the Result pattern a try—it’s a small change that can make a big difference.